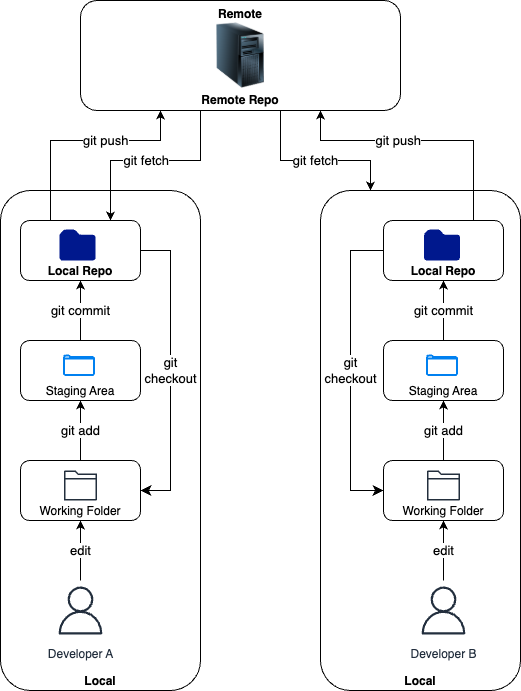
Git is a distributed version control system that helps developers track changes in source code, collaborate with others, and manage projects efficiently. It was created by Linus Torvalds in 2005 to support the development of the Linux kernel and has since become one of the most widely used tools in software development.
Key Features of Git:
1. Version Control: Tracks changes to files over time, enabling you to restore previous versions or compare changes.
2. Distributed: Every developer has a full copy of the entire repository (history and all), which makes it faster and allows for offline work.
3. Branching and Merging: Developers can create branches for new features or bug fixes, work on them independently, and merge them back into the main branch.
4. Collaboration: Allows multiple developers to work on the same project without overwriting each other’s changes.
5. Efficiency: Optimized for speed and performance with low overhead.
Core Concepts in Git:
1. Repository (Repo):
• A directory containing your project files and the entire history of changes.
• Initialized with git init.
2. Commit:
• A snapshot of changes in your code.
• Created using git commit.
3. Branch:
• A parallel line of development.
• The default branch is usually main or master.
4. Merge:
• Combines changes from one branch into another, often from a feature branch into the main branch.
5. Pull and Push:
• Pull: Updates your local repository with changes from a remote repository.
• Push: Uploads your changes from the local repository to a remote repository.
6. Remote:
• A version of your repository stored on a server (e.g., GitHub, GitLab, Bitbucket).
• Added with git remote add.
Basic Git Commands:
Command Description
Command | Description |
git init | Initialize a new Git repository. |
git clone <url> | Clone an existing remote repository. |
git status | Show the status of changes in the working directory. |
git add <file> | Stage changes for the next commit. |
git commit -m “<msg>” | Commit the staged changes with a message. |
git branch | List branches or create a new branch. |
git checkout <branch> | Switch to a different branch. |
git merge <branch> | Merge a branch into the current branch. |
git pull | Fetch and merge changes from a remote repository. |
git push | Push your changes to a remote repository. |
git log | View commit history. |
Git Workflow:
1. Clone or Initialize a Repository:
• Clone: git clone <repository_url>
• Initialize: git init
2. Make Changes:
• Edit files as needed.
3. Stage Changes:
• Add modified files to the staging area using git add.
4. Commit Changes:
• Save changes to the repository with git commit.
5. Sync with Remote:
• Push changes to a remote repository using git push.
• Pull updates from the remote repository with git pull.
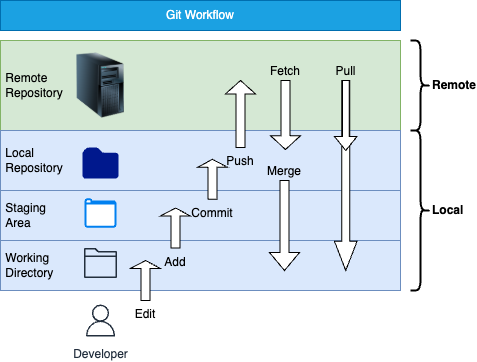
GitHub Integration:
GitHub is a popular hosting service for Git repositories, offering features like pull requests, issue tracking, and CI/CD pipelines.
1. Host Repositories: Store your projects and collaborate with others.
2. Pull Requests: Review and discuss code changes before merging.
3. Issue Tracking: Track bugs, tasks, and feature requests.
4. CI/CD Pipelines: Automate testing and deployment.
Best Practices:
1. Commit Often: Make small, logical commits with descriptive messages.
2. Use Branches: Separate development tasks to avoid conflicts.
3. Pull Frequently: Sync changes to avoid merge conflicts.
4. Resolve Conflicts Carefully: Use tools like git merge or git rebase.
5. Document Workflow: Define a branching strategy (e.g., Git Flow or trunk-based development).