Encapsulation is one of the four fundamental principles of Object-Oriented Programming (OOP), along with abstraction, inheritance, and polymorphism.
Encapsulation is the mechanism of bundling variables(data) and methods (functions) that operate on those variables into a single unit, called a class.
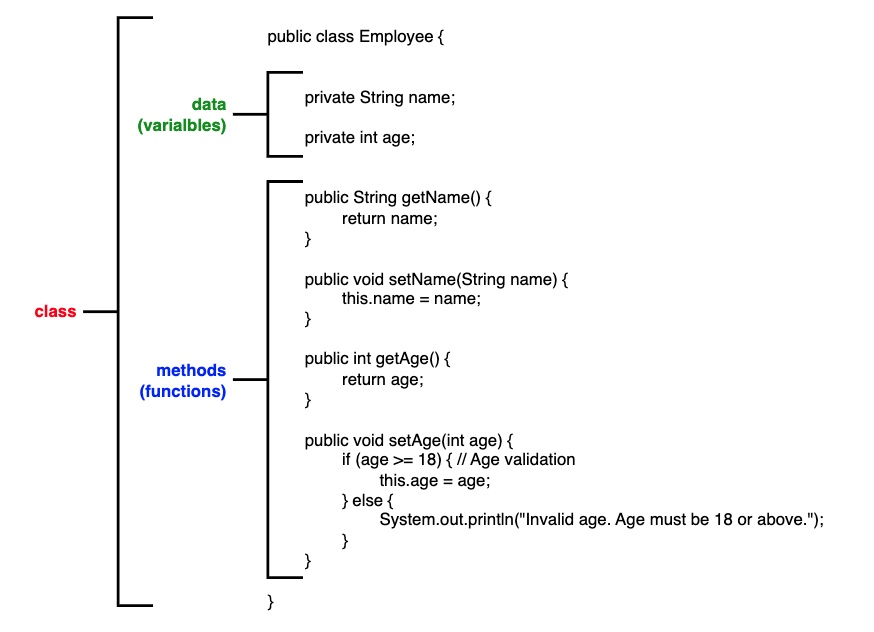
How encapsulation works and benefits:
- Data Hiding: Encapsulation promotes data hiding. By declaring class members as private, you restrict direct access to them from outside the class. This prevents accidental or intentional modification of the data, ensuring data integrity.
- Access Control: To allow controlled access to private members, you can create public methods (often called “getters” and “setters”) that provide read and write access to the data. These methods can include validation logic, ensuring that only valid values are assigned to the data.
- Increased Maintainability: Encapsulation makes code more maintainable. If you need to change how the data is stored or processed internally, you only need to modify the class’s methods, without affecting other parts of the program.
- Improved Reusability: Encapsulated classes are more reusable because they can be treated as self-contained units. You can easily use them in different parts of your program or even in other programs.
How encapsulation is implemented:
- In most OOP languages, encapsulation is implemented through classes and objects.
- Access Specifiers (or Modifiers), such as “private”, “protected”, and “public”, are used to control access to variables and methods.
- “public” allows elements to be accessible from any other class in the application, regardless of the packages. Accessible from everywhere.
- “private” restricts access to the elements only within the class they are declared. Accessible within the same class only.
- “protected” allows access within the same package or in subclass, which might be in different packages. Accessible by the classes of the same package and the subclasses residing in any package.
- default (no modifier specified) accessible by the classes of the same package.
Java Example:
public class Employee {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
if (age >= 18) { // Age validation
this.age = age;
} else {
System.out.println("Invalid age. Age must be 18 or above.");
}
}
}
In this example:
- name and age are private attributes, restricting direct access.
- getName() and setName() provide controlled access to the name attribute.
- getAge() and setAge() provide controlled access to the age attribute, including age validation.
By encapsulating data and methods within the Employee class, we ensure that the data is protected and can only be accessed and modified through the provided methods.